API Design 101: From Basics to Best Practices
API Design 101: From Basics to Best Practices
In this deep dive, we’ll go through the API design, starting from the basics and advancing towards the best practices that define exceptional APIs.
As a developer, you’re likely familiar with many of these concepts, but I’ll provide a detailed explanation to deepen your understanding.
API Design: An E-commerce Example
Let’s consider an API for an e-commerce platform like Shopify, which, if you’re not familiar with is a well-known e-commerce platform that allows businesses to set up online stores.
In API design, we’re concerned with defining the inputs (like product details for a new product) and outputs (like the information returned when someone queries a product) of an API.
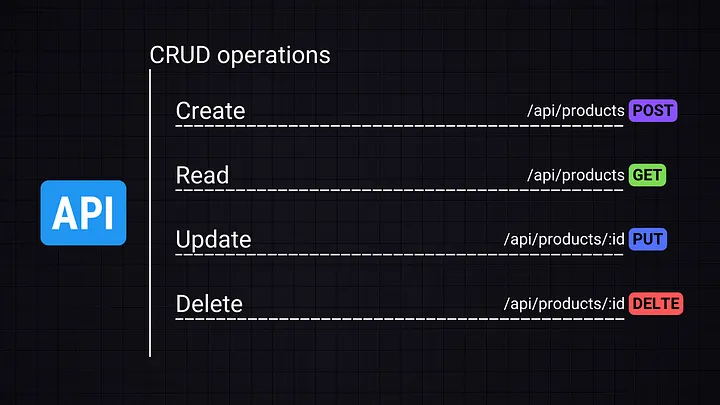
For example, to add a new product (Create), you would make a POST request to /api/products
where the product details are sent in the request body.
To retrieve products (Read), you need to fetch data with a GET request from /products
.
For updating product information (Update), we use PUT or PATCH requests to /products/:id
, where id is the id of a product we need to update.
Removing is similar to updating; we make a DELETE request to /products/:id
where id is the product we need to remove (Delete).
Communication Protocol and Data Transport Mechanism
Another part is to decide on the Communication Protocol that will be used, like HTTP, WebSockets, etc, and the Data Transport Mechanism: JSON, XML, or Protocol Buffers.
This is the case with RESTful APIs, but we also have GraphQL or gRPC paradigms
API Paradigms
APIs come in different paradigms, each with its own set of protocols and standards.
REST (Representational State Transfer)
Pros: Stateless: Each request from a client to a server must contain all the information needed to understand and complete the request. Uses standard HTTP methods (GET, POST, PUT, DELETE). Easily consumable by different clients (browsers, mobile apps).
Cons: This can lead to over-fetching or under-fetching of data- because More endpoints may be required to access specific data.
Features: Supports pagination, filtering (limit
, offset
), and sorting. Uses JSON for data exchange.
GraphQL
Pros: Allows clients to request exactly what they need, avoiding over-fetching and under-fetching. Strongly typed schema-based queries.
Cons: Complex queries can impact server performance. All requests are sent as POST requests.
Features: Typically responds with HTTP 200 status code, even in case of errors, with error details in the response body.
GRPC (Google Remote Procedure Call)
Pros: Built on HTTP/2, it provides advanced features like multiplexing and server push. Uses Protocol Buffers, a language-neutral, platform-neutral, extensible way of serializing structured data. Efficient in terms of bandwidth and resources, especially suitable for microservices.
Cons: Less human-readable compared to JSON. Requires HTTP/2 support.
Features: Supports data streaming and bi-directional communication. Ideal for server-to-server communication.
Relationships in API Design
In an e-commerce setting, you might have relationships like the user to orders, orders to products, etc.
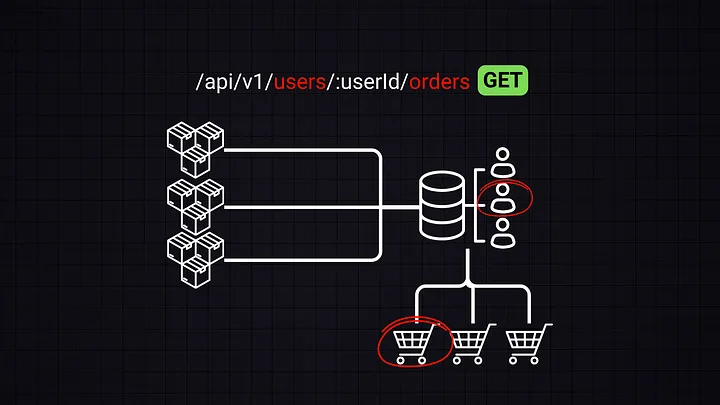
Designing endpoints to reflect these relationships is important. For example, in this scenario GET /users/{userId}/orders
should fetch orders for a specific user.
Queries, Limit, and Idempotence of GET Requests
Common queries also include limit
and offset
for pagination or startDate
and endDate
for filtering products within a certain date range. This allows users to retrieve specific sets of data without overwhelming the system or the user with too much information at once.
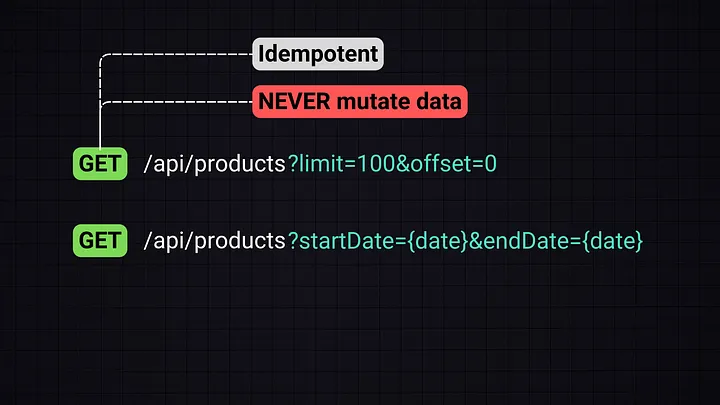
A well-designed GET request is idempotent, meaning calling it multiple times doesn’t change the result.
GET requests should never mutate data. They are meant only for retrieval.
Backward Compatibility and Versioning:
When modifying endpoints, it’s important to maintain backward compatibility. This means ensuring that changes don’t break existing clients.
Versioning: Introducing versions (like /v2/products
) is a common practice to handle significant changes.
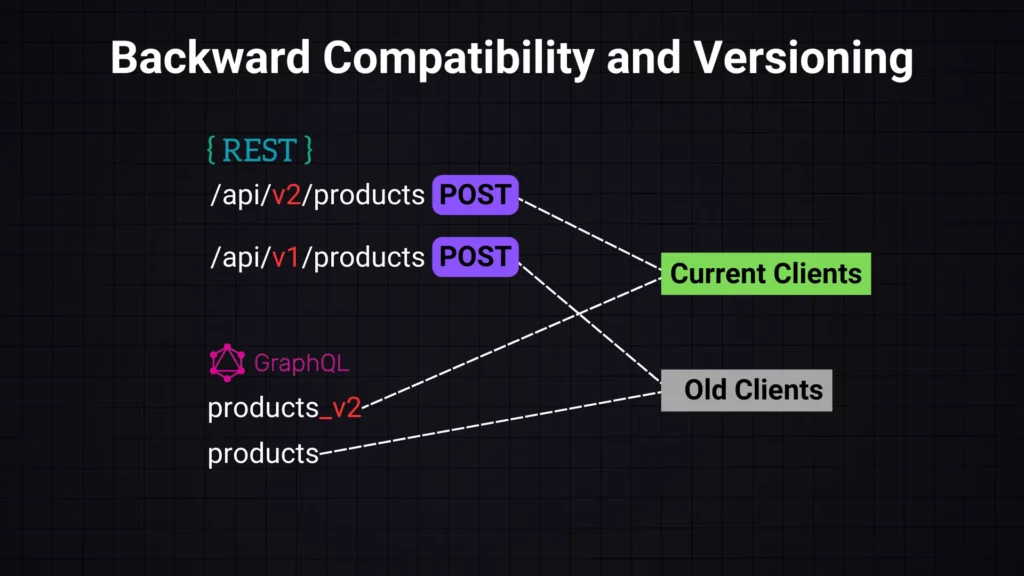
In the case of GraphQL, adding new fields (v2 fields) without removing old ones helps in evolving the API without breaking existing clients.
Rate Limitats and CORS
Another best practice is to set rate limitations. This is used to control the number of requests a user can make in a certain timeframe. This is crucial for maintaining the reliability and availability of your API. It also prevents the API from DDoS attacks.
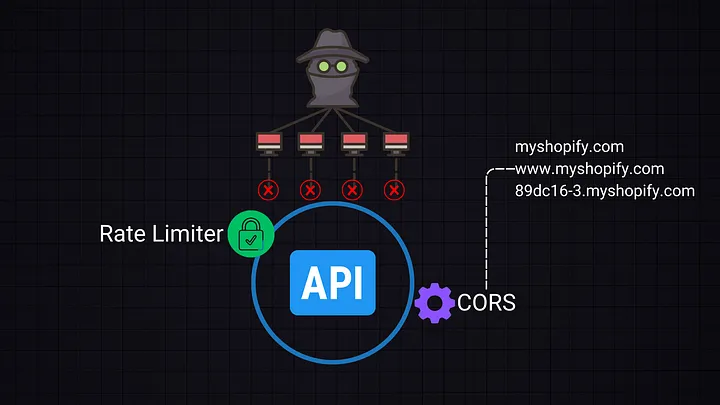
Common practice is to also set CORS settings Cross-Origin Resource Sharing (CORS) settings are important for web security. They control which domains can access your API, preventing unwanted cross-site interactions.
Exploring the Versatility of HTTP Methods
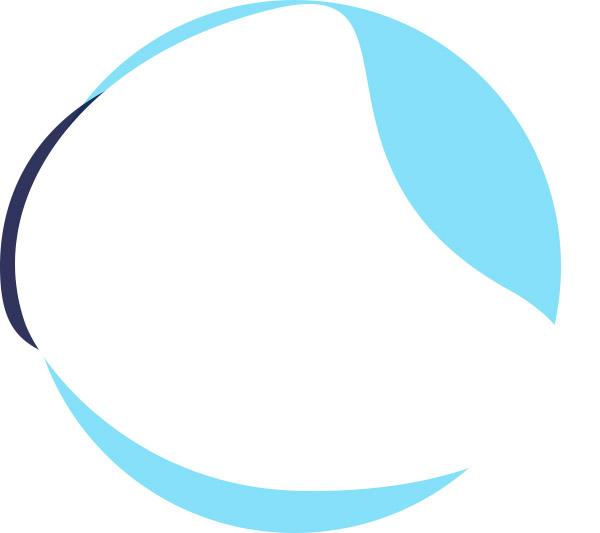
Exploring the Versatility of HTTP Methods
Although most people only use GET, POST, PUT, and DELETE when working with HTTP, there are actually 39 different actions, or “verbs”, in the official list. In this post, we’ll look at 9 of the most popular ones.
Gets data from a server.
Sends data to a server to create something new.
Used to update an existing resource on the server or create it if it doesn’t exist. For example, a client might use a PUT request to send updated information about a product to the server, overwriting the existing data or creating a new product entry if it doesn’t already exist.
When you update your profile on a social media site by only changing your profile picture, the server may use the PATCH method to make this modification.
If you want to delete a message or comment you posted on an online forum, a DELETE request can be used to perform this deletion.
Browsers often use HEAD to check if a webpage has changed since the last visit. This helps determine whether to download the full page or if the cached version is still valid.
This method is used by clients to determine which methods are allowed on a resource. For example, a client can send an OPTIONS request to an API to find out which methods are supported by that API.
TRACE is not commonly used in everyday scenarios. It’s mainly used for advanced debugging and tracking the routing of requests.
The CONNECT method is primarily used to establish SSL/TLS connections through a proxy. It’s mostly transparent to most users and is handled by the browser or web client.
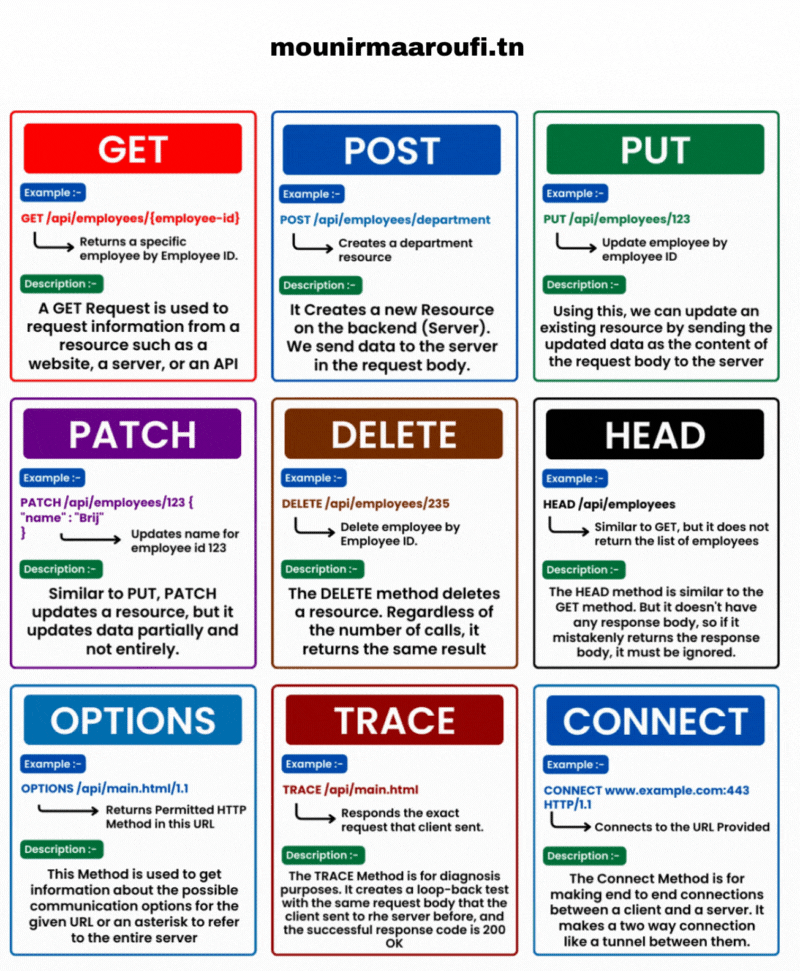
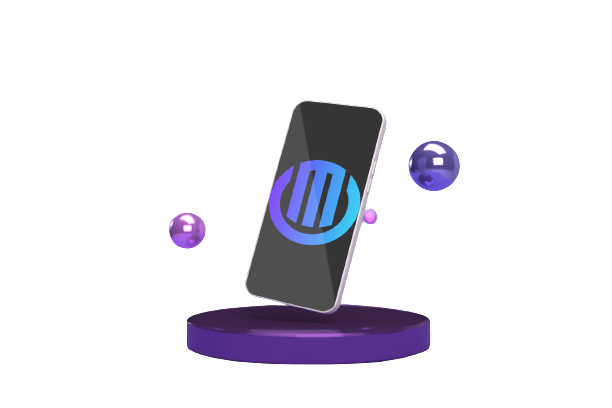
How GIT Works ?
Git’s popularity is undeniable, as it has become an essential tool for version control and collaboration, making it nearly impossible to ignore its significance in modern coding workflows.
Demystifying RESTful APIs: Your Gateway to Modern Web Development
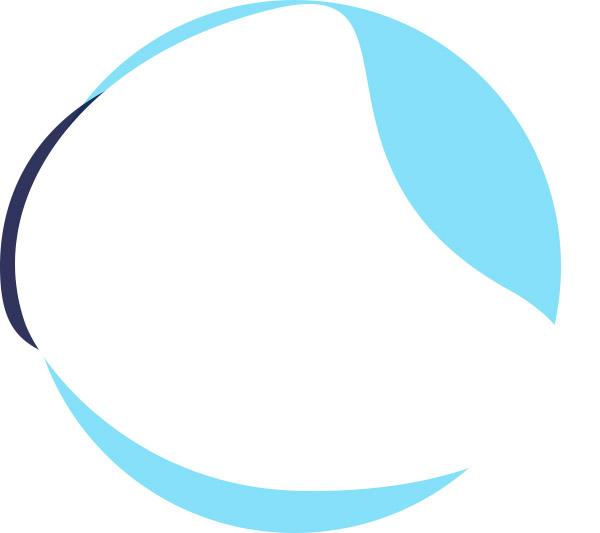
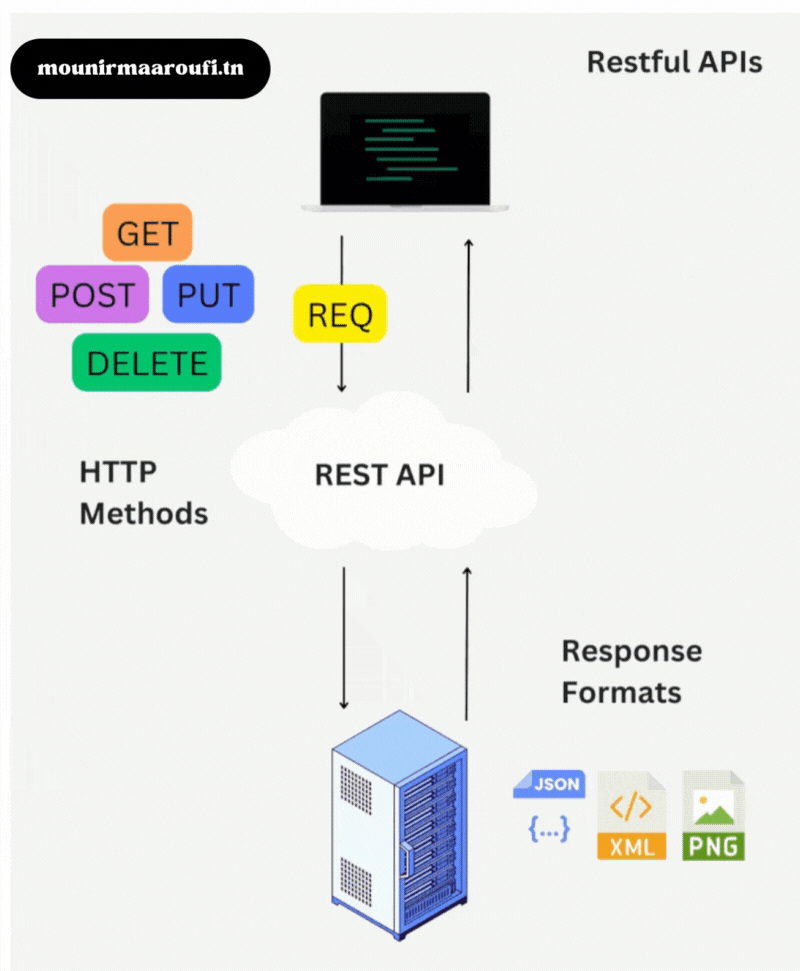
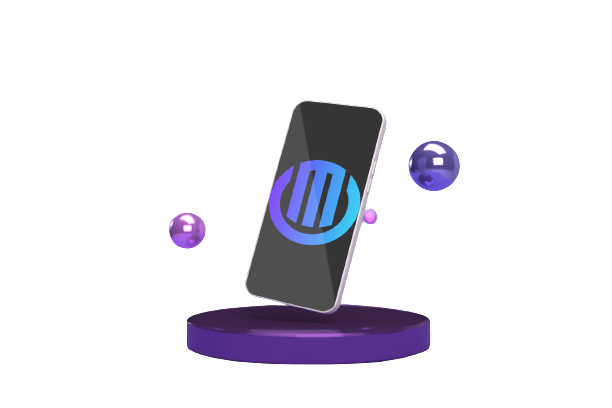
Demystifying RESTful APIs: Your Gateway to Modern Web Development
RESTful API, or Representational State Transfer API, serves as the backbone of modern web applications, acting as a virtual file cabinet that stores and manages data on the vast expanse of the internet. Understanding how RESTful APIs work is fundamental for developers and businesses looking to harness the power of the web.
🔗 REST, short for Representational State Transfer, employs standard HTTP methods to interact with data. Let’s break down these methods to help you grasp their significance:
- Let’s say you want to retrieve information about users from your website. You’d send a GET request like this:
- GET
https://www.mounirmaaroufi.com/users
To add new data, like a blog post, you’d use a POST request:
- POST
https://www.mounirmaaroufi.com/blog
For updating data, such as editing a blog post, the PUT request is used:
- PUT
https://www.mounirmaaroufi.com/blog/{blog_id}
When it’s time to remove data, like deleting a blog post, a DELETE request is employed:
- DELETE
https://www.mounirmaaroufi.com/blog/{blog_id}
The Simplest way to Make a back to Top Button
☑️ 𝗧𝗵𝗲 𝗦𝗶𝗺𝗽𝗹𝗲𝘀𝘁 𝘄𝗮𝘆 𝘁𝗼 𝗠𝗮𝗸𝗲 𝗮 𝗕𝗮𝗰𝗸 𝘁𝗼 𝗧𝗼𝗽 𝗕𝘂𝘁𝘁𝗼𝗻
✔️ 𝙏𝙝𝙚 𝙒𝙞𝙣𝙙𝙤𝙬.𝙨𝙘𝙧𝙤𝙡𝙡𝙏𝙤() 𝘮𝘦𝘵𝘩𝘰𝘥 𝘴𝘤𝘳𝘰𝘭𝘭𝘴 𝘵𝘩𝘦 𝘸𝘪𝘯𝘥𝘰𝘸 𝘵𝘰 𝘢 𝘱𝘢𝘳𝘵𝘪𝘤𝘶𝘭𝘢𝘳 𝘱𝘭𝘢𝘤𝘦 𝘪𝘯 𝘵𝘩𝘦 𝘥𝘰𝘤𝘶𝘮𝘦𝘯𝘵.
✔️ 𝘄𝗶𝗻𝗱𝗼𝘄.𝘀𝗰𝗿𝗼𝗹𝗹𝗧𝗼(𝘅-𝗰𝗼𝗼𝗿𝗱, 𝘆-𝗰𝗼𝗼𝗿𝗱)
⦿ 𝙭-𝙘𝙤𝙤𝙧𝙙 𝘪𝘴 𝘵𝘩𝘦 𝘱𝘪𝘹𝘦𝘭 𝘢𝘭𝘰𝘯𝘨 𝘵𝘩𝘦 𝘩𝘰𝘳𝘪𝘻𝘰𝘯𝘵𝘢𝘭 𝘢𝘹𝘪𝘴 𝘰𝘧 𝘵𝘩𝘦 𝘥𝘰𝘤𝘶𝘮𝘦𝘯𝘵 𝘵𝘩𝘢𝘵 𝘺𝘰𝘶 𝘸𝘢𝘯𝘵 𝘵𝘰 𝘣𝘦 𝘥𝘪𝘴𝘱𝘭𝘢𝘺𝘦𝘥 𝘪𝘯 𝘵𝘩𝘦 𝘶𝘱𝘱𝘦𝘳 𝘭𝘦𝘧𝘵.
⦿ 𝙮-𝙘𝙤𝙤𝙧𝙙 𝘪𝘴 𝘵𝘩𝘦 𝘱𝘪𝘹𝘦𝘭 𝘢𝘭𝘰𝘯𝘨 𝘵𝘩𝘦 𝘷𝘦𝘳𝘵𝘪𝘤𝘢𝘭 𝘢𝘹𝘪𝘴 𝘰𝘧 𝘵𝘩𝘦 𝘥𝘰𝘤𝘶𝘮𝘦𝘯𝘵 𝘵𝘩𝘢𝘵 𝘺𝘰𝘶 𝘸𝘢𝘯𝘵 𝘵𝘰 𝘣𝘦 𝘥𝘪𝘴𝘱𝘭𝘢𝘺𝘦𝘥 𝘪𝘯 𝘵𝘩𝘦 𝘶𝘱𝘱𝘦𝘳 𝘭𝘦𝘧𝘵.
Check full code implementation with button in the comment section
_________________________________
😃 𝙉𝙞𝙘𝙚 𝙢𝙖𝙣, 𝙘𝙝𝙚𝙚𝙧𝙨 🥂
𝗜𝗳 𝘆𝗼𝘂 𝗹𝗲𝗮𝗿𝗻 𝗮 𝗻𝗲𝘄 𝗰𝗼𝗻𝗰𝗲𝗽𝘁 𝘁𝗼𝗱𝗮𝘆 , 𝘀𝗵𝗼𝘄 𝗺𝗲 𝘆𝗼𝘂𝗿 𝘀𝘂𝗽𝗽𝗼𝗿𝘁 , 𝗵𝗶𝘁 𝘁𝗵𝗲 𝗹𝗶𝗸𝗲(👍) 𝗯𝘂𝘁𝘁𝗼𝗻 ❤️
𝗜 𝗵𝗮𝘃𝗲 𝗯𝗲𝗲𝗻 𝗽𝗼𝘀𝘁𝗶𝗻𝗴 𝗮𝗯𝗼𝘂𝘁 𝘄𝗲𝗯 𝗱𝗲𝘃 𝘁𝗿𝗶𝗰𝗸𝘀,
𝗜 𝗮𝗰𝗰𝗲𝗽𝘁 𝗲𝘃𝗲𝗿𝘆 𝗰𝗼𝗻𝗻𝗲𝗰𝘁𝗶𝗼𝗻 𝗿𝗲𝗾𝘂𝗲𝘀𝘁,😃 𝗹𝗲𝘁’𝘀 𝗰𝗼𝗻𝗻𝗲𝗰𝘁 Mounir Maaroufi 🌍
How to Become A Full-Stack Developer
Blog
How to Become A Full-Stack Developer?
After all, a quick Google search for “full stack development” renders an impossibly long list of acronyms: HTML, CSS, JS, MySQL, and PHP, just to name a few. At heart, full stack developers are highly versatile jacks-of-all-trades in an industry that demands comprehensive programming knowledge.
Take a breath: learning how to become a full stack web developer isn’t as difficult or time-consuming as you initially might think. In this article, we’ll walk you through the basics of full stack development, what you need to learn, and how you can prepare yourself for a full-blown career in development.
Wondering what’s next? Here’s a sneak peek at our six steps to becoming a full stack developer:
- Identify The Skills You Need to Learn
- Determine Your Ideal Timeline
- Start Learning in a Format That Suits You
- Begin Building Your Portfolio
- Start the Job Search
- Ace the Interview
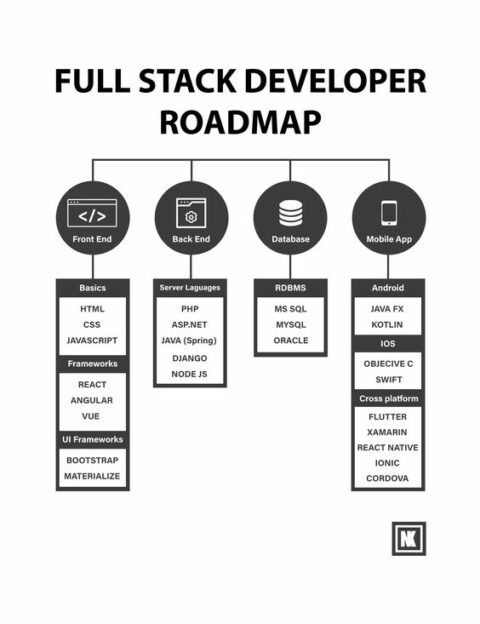
Web Development Process
Blog
Web Development Process
Every web development project has to go through certain steps that take the project from initiation to completion. Here Mounir Maaroufi has shared an Infographic for Web Development Process.
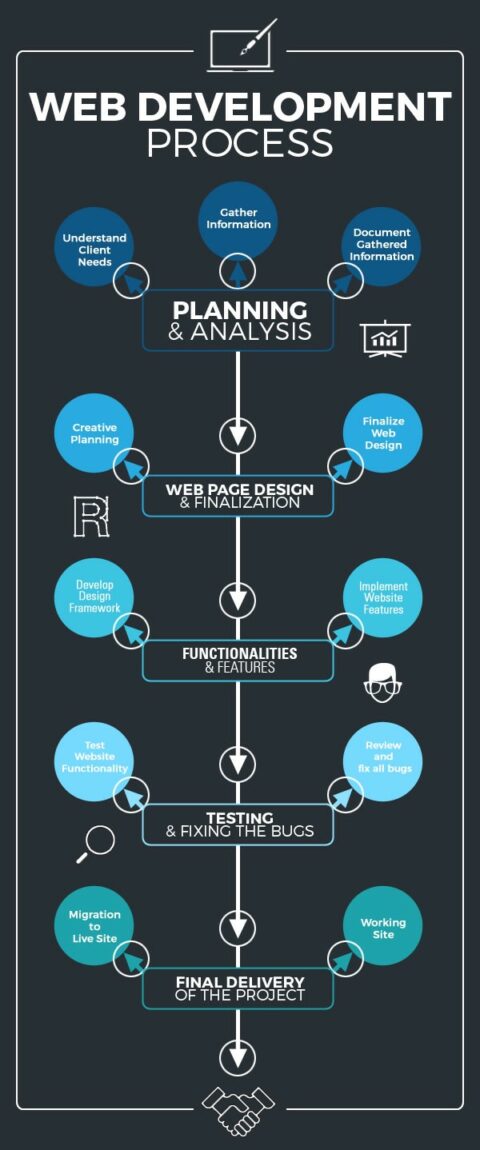